Software Design Patterns
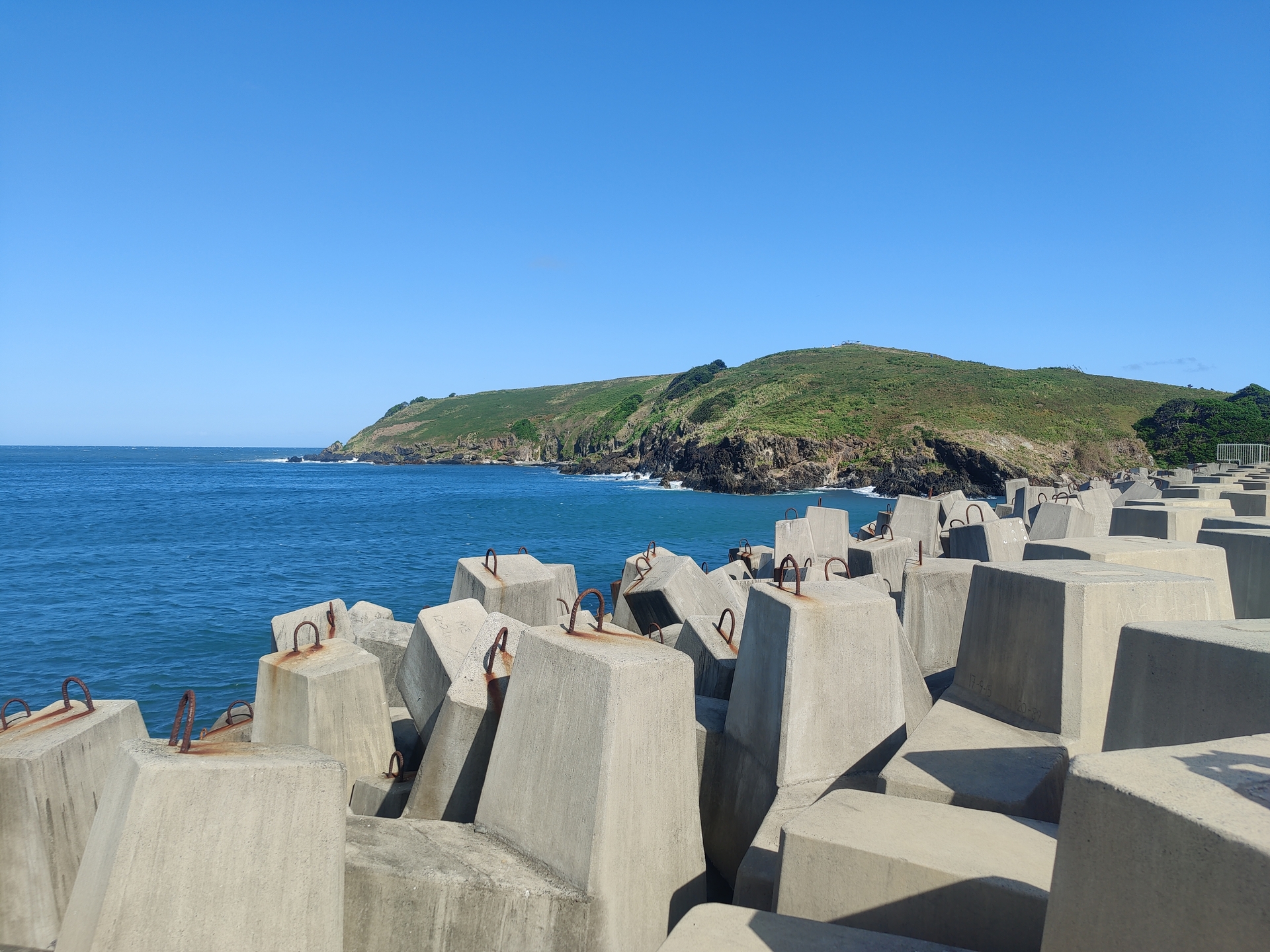
What is a design pattern?
Design patterns are conventional solutions to typical problems that arise in software design. They are like a blueprint for solutions to typical problems that you can customize. The patterns is only general concepts which you can implement specifically for your use case/problem.
Why should I learn patterns?
The good thing about design patterns is that they are tried and tested solutions to common problems. This allows you to solve problems you encounter in your programs in an efficient way without having to reinvent the wheel. If you and your team members know about patterns, it can help making the communication more efficient.
There are also some drawback about using patterns. When you know about the software patterns it can be tempting to try to implement a pattern where it is not needed or the best solution. Another drawback is to try to implement a pattern to literally, and not customizing it to the context of the project.
Classification of patterns
Software patterns is often classified in three different categories: creational patterns, structural patterns and behavioral pattern. Creational patterns describes methods to create objects that improve code reuse and flexibility. Structural patterns provide patterns for how to build objects and classes into larger structures, while making them adaptable and efficient. Behavioral patterns describes the patterns used for efficient communication and the delegating of responsibility amongst objects.
Creational design patterns
Factory method
Factory method provides an interface for creating objects in a superclass. The subclasses can still choose which specific objects are being created. Instead of creating the objects directly using the new
keyword, the objects are created using a factory method.
The factory method moves the creation of objects into the subclasses. The subclasses must implement a factory method which creates the objects using the new
method. All subclasses new to create an object following a common interface.
Singleton
Singleton is a creational pattern which allows that a class only has one instance. To create a singleton class, the default constructor of a class needs to be private. This ensures that other classes can't call the new
method on the singleton class. Instead of using the default constructor, create a static creation method. This method calls the private constructor if there doesn't exist an object, otherwise it returns the already existing cached object.
The problems that the singleton class solves is that it ensures that a class only has a single instance and it can provide a global access point to that instance.
Builder
Builder is a creational pattern that allows you to create complex objects step by step. This can also allow for using the same construction code to implement different types and representations.
What is the problem being solved using the builder class?
If there exist an class that can have a lot of variations, the constructor can become quite large and messy. For instance, imagine a Car
class with a lot of options for extra equipment (ABS, heated seat, rear view camera, etc.). Instead of having a big car constructor with all the options, it can be convenient with a builder class. The builder class can have a series of methods which constructs different variations of the car. For instance buildFrame
, addWheels
, addBrakes
, addRearViewCamera
, etc...
It is also possible to have several builder class, which implements the same steps, but in a different manner. The factory of a VW isn't the same as a Ferrari, but it has similar steps.
Structural patterns
Adapter
Adapter pattern is a pattern which allows two classes with different interfaces to communicate. The adapter work as a translator between the classes such that they can work together. For instance, if a class outputs XML and the other class requires JSON input. Then you need an adapter to convert the XML to JSON.
Proxy
Proxy is a structural pattern that let's you control access to an object by creating a substitute or placeholder. If there exist an service or resource which is not always running, the proxy can handle the initialization of the service. Then the initialization code don't need to be duplicated in all places which uses the service.
The proxy pattern suggest that you create a new proxy class with the same interface as the original object. Then you can use the proxy object instead of the original object in your program. The proxy then delegates the work to the original object.
The advantage of using a proxy is that you can control access to the original object and a proxy can work even if the original object is down.
Behavioral patterns
State
The state pattern handles an objects behavior when the internal state changes. For instance, there is certain behavior that is not allowed when an object is in a specific state.
The state pattern is closely connected to finite-state machine. This tells us that there is only a certain number of states a program can be in. The states behave differently, and not all states can transition between each other.
To create the logic which encapsulates the rules for transitions between states, and the behavior of the state it is common to conditional statements (if or switch or pattern matching). If there is a lot of states, this logic can become quite big and difficult to maintain.
The state pattern solves this by implementing classes for each state. Each state class handles its own behavior and the logic for transitioning into a new state. The original object, also known as context, change states by replacing the active state object with a new state object. All the state objects need to follow a common interface.
Strategy
The strategy pattern allows family of algorithms to be grouped under an abstraction. This allows the runtime to switch between algorithms. The strategy pattern is quite similar to the state pattern. Instead of handling different state, it handles different algorithms or strategies.
For instance, Google Maps have an option for navigating using car, walking, public transport, etc... This options uses different algorithms, but can be used in the same client.
The strategy pattern uses an generic interface for the different strategies. The original class becomes independent of the concrete strategies. The original class has a field for storing a strategy object. Then specific strategy object is responsible for executing the algorithm. The client is the one responsible for choosing the correct algorithm.
An advantage of using the strategy pattern is that you can add additional algorithms in the future without cluttering the original class.
Observer
Observer is a pattern describing a subscription mechanism which allows multiple object to be notified when an object they are observing changes.
The object that is observed is often called subject, or publisher. The object that is notified when changes happen are called a subscriber. YouTube is an example of the observer pattern, where every time a channel publishes a new video, the followers are notified.
The publisher class should have methods for adding and removing subscribers and notifying all the subscribers. All the subscribers should have the same interface, and method which the publisher can call to notify of the change. Often the publisher has a list of subscribers, and loops through the subscribers and call the subscribers update
method to notify of the publishers change.